Arduino Temperature and Humidity monitor Tutorial with DHT11 (or DHT22) sensor
This Post will demonstrate how to use DHT Series to measure the Temperature & Humidity of your room, office, warehouse, kitchen and where you want to messure the temperature & Humidity 🙂
Hardware Required:
1x Arduino Uno
1x DHT11 Temperature & Humidity Sensor or 1x DHT22 Temperature & Humidity Sensor
Complete Video Instructions:
Pin Layout:
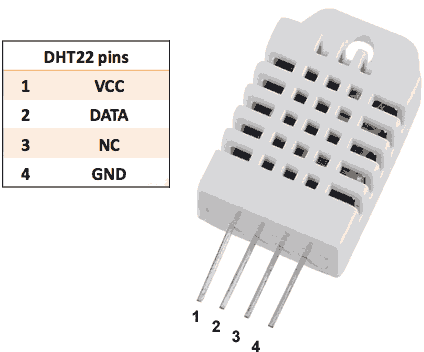
DHT11 VS DHT22:
Specification | DHT11 | DHT22 |
Temperature Range | 0 – 50 °C / ±2°C | -40 – 125 °C / ±0.5°C |
Humidity Range | 20 – 80% / ± 5% | 0 – 100% / ± 2-5% |
Sampling Rate | 1 Hz (one reading every second) | 0.5 Hz(one reading every two seconds) |
Body Size | 15.5mm x 12mm x 5.5mm | 15.1mm x 25mm x 7.7mm |
Operating Voltage | 3-5 V | |
Max Current During Measuring | 2.5 mA | 2.5 mA |
GitHub DHT Library Link Click Here
Code:
/*
* How to use the DHT-22 sensor with Arduino uno Temperature and humidity sensor
* Writer OR97.com
* Instructions Link:https://www.or97.com/arduino-temperature-and-humidity-monitor-tutorial-with-dht11-or-dht22-sensor
*/
//Libraries
#include <DHT.h>;
//Constants
#define DHTPIN 7 // what pin we’re connected to
#define DHTTYPE DHT22 // DHT 22 (AM2302)
DHT dht(DHTPIN, DHTTYPE); //// Initialize DHT sensor for normal 16mhz Arduino
//Variables
int chk;
float hum; //Stores humidity value
float temp; //Stores temperature value
void setup()
{
Serial.begin(9600);
dht.begin();
}
void loop()
{
delay(2000);
//Read data and store it to variables hum and temp
hum = dht.readHumidity();
temp= dht.readTemperature();
//Print temp and humidity values to serial monitor
Serial.print(“Humidity: “);
Serial.print(hum);
Serial.print(” %, Temp: “);
Serial.print(temp);
Serial.println(” Celsius”);
delay(10000); //Delay 10 sec.
}